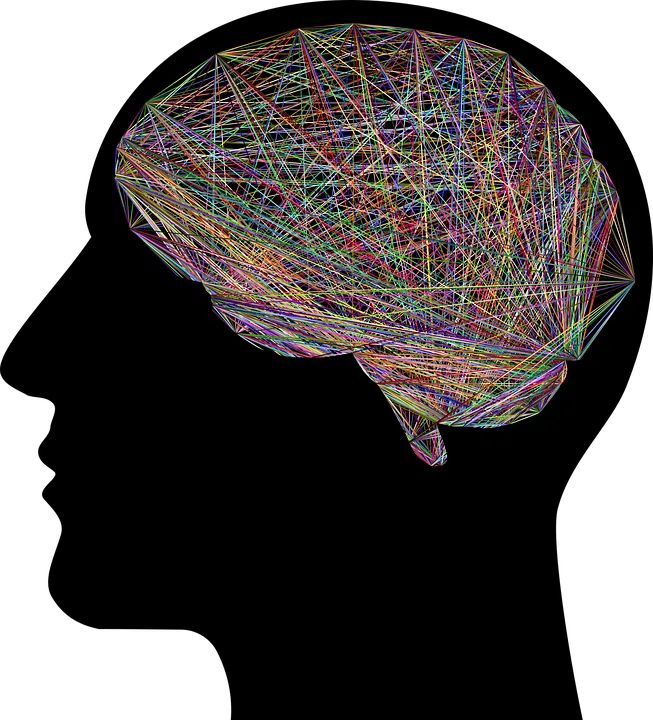
A Graph Neural Network (GNN) is a type of neural network that is designed to work with graph-structured data, such as data that represents relationships between entities. GNNs are typically used for tasks such as node classification, link prediction, and graph classification.
A key component of a GNN is the message-passing mechanism, which allows information to flow between the nodes in a graph. The message-passing mechanism typically involves the use of a “message function” that takes as input the current node’s feature vector and the feature vectors of its neighboring nodes, and produces an updated feature vector for the current node.
The use of “picture codes” in GNNs refers to the representation of graphs as images. In this approach, nodes in a graph are represented as pixels in an image, and edges are represented by the presence of a colored link between those pixels. This approach allows GNNs to leverage pre-trained convolutional neural networks (CNNs) to perform tasks such as node classification and link prediction.
For example, in a node classification task, an image of the graph would be input to a pre-trained CNN, which would then output a feature vector for each node in the graph. These feature vectors could then be used as input to a fully connected neural network to classify each node in the graph.
In a link prediction task, a pair of nodes, say A and B are considered, and a sub-graph formed by those nodes and its neighours, where the edges between the nodes A and B can be labeled as present or absent. Then this sub-graph is transformed into an image and pass through a pre-trained CNN which would output a probability of the edge between A and B.
To sum up GNNs and picture codes, it is a way to combine the power of CNN to analyze graph based data by representing the graph as an image, where each node is a pixel, and edges are represented as the link between pixels with different color channels.
Mechanics Of GNNs
The mechanism of a Graph Neural Network (GNN) involves updating the feature vectors of nodes in a graph through message passing. The message passing process can be broken down into two main steps:
- Neighbor Aggregation: In this step, the feature vectors of a node’s neighboring nodes are aggregated to produce a new representation for the current node. This is typically done by applying a “message function” to the feature vectors of the neighboring nodes. The message function can be a simple operation such as averaging, or a more complex operation such as a neural network.
- Node Update: In this step, the new representation produced by the neighbor aggregation step is combined with the current node’s feature vector to produce an updated feature vector. This can be done by concatenating the two vectors, or by applying another neural network to the new representation and current feature vector.
These two steps are typically repeated multiple times, with each iteration updating the feature vectors of all nodes in the graph. The output of the GNN is then typically the final feature vectors of all nodes, which can be used for tasks such as node classification and link prediction.
When it comes to picture codes, the mechanism is slightly different, it works by first representing the graph as an image. where each node is represented as a pixel, and edges are represented as links between pixels with different color channels. This image is then input to a pre-trained convolutional neural network (CNN), which produces a feature vector for each node in the graph. The feature vectors can then be used as input to a fully connected neural network or another GNN.
In summary, a GNN uses message passing to aggregate information from a node’s neighboring nodes and update the node’s feature vector. By representing graphs as images and using pre-trained CNNs, picture codes in GNNs allows to effectively analyze graph structured data and perform tasks such as node classification and link prediction.
Here’s an example of how a GNN might be used to perform node classification on a small graph:
# Import necessary libraries
import numpy as np
import torch
from torch import nn
# Define the graph
# The graph has 3 nodes and 2 edges
# Node 0 is connected to node 1
# Node 1 is connected to nodes 0 and 2
# Node 2 is connected to node 1
adj = [[0, 1, 0],
[1, 0, 1],
[0, 1, 0]]
# Define the feature vectors for each node
# Each node has a feature vector of length 2
x = torch.tensor([[0.2, 0.5],
[0.1, 0.7],
[0.4, 0.8]])
# Define the message function
# This is a simple linear transformation of the neighboring nodes' feature vectors
class MessageFunction(nn.Module):
def __init__(self):
super().__init__()
self.fc = nn.Linear(2, 2)
def forward(self, x, adj):
x = self.fc(x)
x = torch.matmul(adj, x)
return x
# Define the update function
# This simply concatenates the current node's feature vector with the message
class UpdateFunction(nn.Module):
def __init__(self):
super().__init__()
def forward(self, x, m):
x = torch.cat([x, m], dim=-1)
return x
# Define the GNN
class GNN(nn.Module):
def __init__(self):
super().__init__()
self.message_function = MessageFunction()
self.update_function = UpdateFunction()
def forward(self, x, adj):
for _ in range(2): # repeat 2 times
m = self.message_function(x, adj)
x = self.update_function(x, m)
return x
# Define the classification layer
# This is a simple linear transformation followed by a softmax
class Classifier(nn.Module):
def __init__(self):
super().__init__()
self.fc = nn.Linear(4, 3)
def forward(self, x):
x = self.fc(x)
x = nn.functional.softmax(x, dim=-1)
return x
# Put it all together
gnn = GNN()
classifier = Classifier()
output = classifier(gnn(x, adj))
print(output)
In this example, the graph is defined as an adjacency matrix adj
, where adj[i][j] = 1
indicates that there is an edge between node i and node j. The feature vectors for each node are stored in a tensor x
.
The message function is implemented as a simple linear transformation followed by a matrix multiplication with the adjacency matrix. The update function concatenates the current node’s feature vector with the message produced by the message function. The GNN applies these two functions in two iterations.
The classification layer is a simple linear transformation followed by a softmax.
GNN takes as input the feature vectors of the nodes and the adjacency matrix and performs message passing to update the feature vectors of the nodes. The output of the GNN is then passed through the classification layer to produce the final output, which in this case is a probability distribution over the possible class labels for each node.
In this example I used a very small graph and a simple architecture, but in real-world applications GNNs can be used to process much larger and more complex graph structures. Also, different types of GNN models, like GCN, GAT, GIN, etc can be used in different applications, each with its own strengths and weaknesses.
As for picture code representation, the graph is represented as an image, where each node is a pixel, and edges are represented as links between pixels with different color channels. Then this image is passed through a pre-trained CNN and features are extracted, these features are then passed through a fully connected neural network or a GNN to perform the respective task.
Keep in mind that this is just an example of how GNNs can be implemented and used, there are many variations and extensions of GNNs that have been proposed in recent research.
There are several types of Graph Neural Networks (GNNs) that have been proposed in recent research. Some of the most popular types include:
- Graph Convolutional Networks (GCN): GCNs extend the idea of convolutional neural networks (CNNs) to graph-structured data. In GCNs, the convolution operation is replaced by a graph convolution operation, which aggregates information from a node’s neighboring nodes to update the node’s feature vector. GCNs are particularly well-suited for semi-supervised node classification tasks.
- Graph Attention Networks (GAT): GATs introduce the concept of attention mechanisms to GNNs. Instead of aggregating information from all neighboring nodes equally, GATs assign a weight to each neighboring node based on its importance for the task at hand. GATs are particularly well-suited for tasks such as node classification and link prediction.
- Graph Isomorphism Networks (GIN): GINs are a type of GNN that are designed to be invariant to permutations of the nodes in a graph. GINs use a neural network to compare the neighborhoods of different nodes, and use the output of this comparison to update the feature vectors of the nodes. GINs are particularly well-suited for tasks such as graph classification and molecule property prediction
- GraphSAGE: It’s a inductive method which allows to handle large graphs with unseen nodes. It generalizes the neighborhood aggregation framework of GCN by allowing an arbitrary function to sample a fixed number of neighbors and aggregate their representations before updating the current node.
- Graph Transformer Networks: These GNN models focus on handling the node dependencies over different scales of the graph, they take advantage of transformer architecture, similar to the ones used in language and vision problems.
There are other models too which are specific to a particular task, each type of GNN is suitable for different kinds of problems and has its own set of advantages and limitations.
Challenges:
representing graphs in a format that is compatible with machine learning models can be a challenge due to the unique structure of graph data. Some of the key challenges include:
- Sparsity: As you mentioned, graphs can be very sparse, which can make it difficult to efficiently represent them using adjacency matrices or other dense representations.
- Permutation invariance: The connectivity of a graph can be represented in many different ways, and there is no guarantee that different representations will produce the same result when used as input to a neural network. This means that the neural network must be able to learn permutation-invariant operations.
- Node and edge feature integration: In many graph-based tasks, both the node and edge features are important for making predictions. Integrating these two types of information can be challenging, as they have different dimensions and structures.
- Handling variable graph sizes: Graphs can have a variable number of nodes and edges, which can make it difficult to design a neural network that can handle inputs of different sizes.
- Scalability: As the number of nodes and edges in a graph increases, the computational and memory requirements of a graph neural network can become prohibitively large.
To overcome these challenges, researchers have proposed different methods for representing graphs, such as graph convolutional networks (GCNs), graph attention networks (GATs), and graph autoencoders. These methods typically involve either transforming the graph into a grid-like representation or using pooling operations to summarize the graph’s structure. Additionally, researchers have proposed different techniques for handling permutation invariance and integrating node and edge features.
Despite the challenges, the use of neural networks on graph-based data has been a very active area of research and has shown promising results in a wide range of tasks including node classification, link prediction.
Real Life Applications:
Graph Neural Networks (GNNs) have been used in a variety of real-world applications, here are some examples:
- Social network analysis: GNNs have been used to analyze social networks, such as predicting the formation of new links between individuals or identifying communities within the network.
- Recommender systems: GNNs can be used to recommend items or content to users based on the graph structure of the data. For example, GNNs have been used to recommend movies or music to users based on their past viewing/listening history and the viewing/listening history of other users in their social network.
- Protein structure prediction: GNNs have been used to predict the 3D structure of proteins from their amino acid sequence. Since proteins can be represented as graphs, where the nodes are the amino acids and the edges are the chemical bonds between them, GNNs can be used to learn the relationship between a protein’s amino acid sequence and its 3D structure.
- Drug discovery: GNNs have been used to predict the potential interactions between different molecules, which can aid in the discovery of new drugs.
- Computer vision: GNNs have been used to improve object detection and segmentation in images by incorporating graph-based representations of the image’s object relations.
- Natural Language Processing: GNNs have been used in many NLP tasks such as relation extraction, sentiment analysis, and text generation by representing the sentence as a graph where words are the nodes and edges represent the relation between them.
- Robotics: GNNs have been used to improve the decision-making abilities of robots in complex, dynamic environments by representing the environment as a graph and using GNNs to analyze the graph and plan the robot’s actions.
These are just a few examples, but GNNs are being used in many different fields because of its ability to handle graph-structured data and make predictions. As the research in this field progresses, we will likely see more and more innovative applications of GNNs.
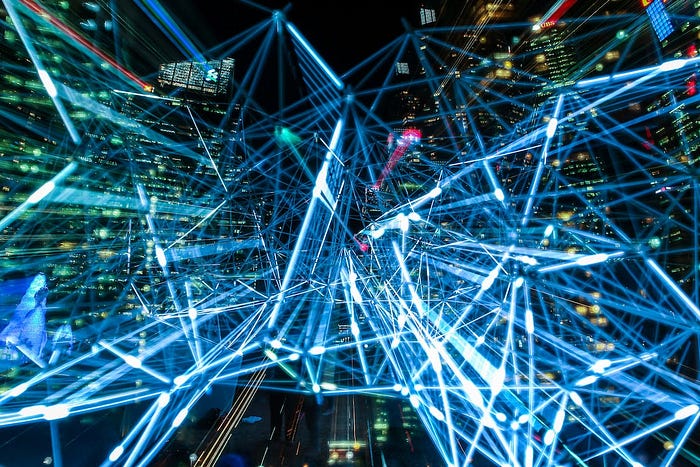
#Gnn
#Neural Networks
#Artificial Intelligence
#Towards Data Science
#Beginners Guide
Leave a Reply
You must be logged in to post a comment.