Lets understand Python “random” Function and difference with “numpy random” funtion
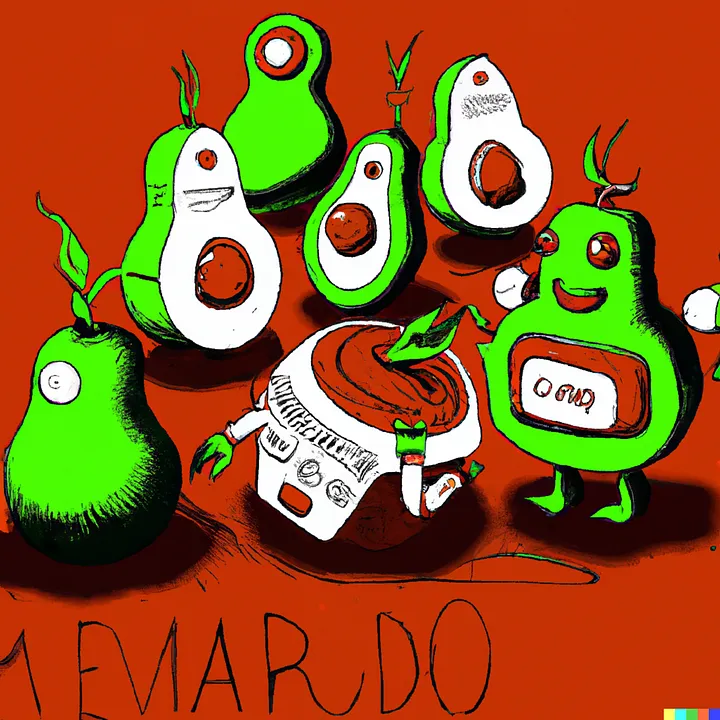
Python has a built-in module called
random
that provides various functions to generate random numbers and perform other random operations. Lets understand it with real examples, that will make it easier to understand.
Below are a few commonly used functions in the random
module:
- random.random(): This function returns a random float in the interval [0.0, 1.0].
2. random.randint(a, b): This function returns a random integer between a and b, inclusive.
3. random.choice(sequence): This function returns a random element from the given sequence.
4. random.shuffle(sequence): This function shuffles the elements of the sequence in place.
5. random.sample(population, k): This function returns a list of k unique elements randomly selected from the population.
Consider a dataset of heights of people in centimeters. We’ll use this dataset to demonstrate how the random module can be used to perform various operations.
import random
# create a dataset of heights of people in centimeters
heights = [170, 175, 180, 185, 190, 195, 200, 205, 210, 215]
# generate a random height from the dataset
random_height = random.choice(heights)
print("Random height:", random_height)
# shuffle the heights in the dataset
random.shuffle(heights)
print("Shuffled heights:", heights)
# generate a random sample of 2 heights from the dataset
random_sample = random.sample(heights, 2)
print("Random sample:", random_sample)
Random height: 170
Shuffled heights: [200, 180, 215, 185, 175, 205, 195, 210, 170, 190]
Random sample: [180, 170]
This example, we first create a dataset of heights of people in centimeters. Then we use the random.choice() function to generate a random height from the dataset. Next, we use the random.shuffle() function to shuffle the heights in the dataset. Finally, we use the random.sample() function to generate a random sample of 2 heights from the dataset.
Let’s see some more Examples!
- Generating random numbers within a range:
import random
# generate a random integer between 1 and 100
random_number = random.randint(1, 100)
print("Random number:", random_number)
# generate a random float between 0.0 and 10.0
random_float = random.uniform(0.0, 10.0)
print("Random float:", random_float)
Random number: 25
Random float: 5.110349141483917
2.Generating random elements from a sequence:
import random
# create a sequence of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# generate a random number from the sequence
random_number = random.choice(numbers)
print("Random number:", random_number)
# generate a random sample of 5 numbers from the sequence
random_sample = random.sample(numbers, 5)
print("Random sample:", random_sample)
Random number: 8
Random sample: [9, 7, 6, 10, 1]
3. Shuffling a sequence:
import random
# create a sequence of numbers
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# shuffle the numbers in the sequence
random.shuffle(numbers)
print("Shuffled numbers:", numbers)
Shuffled numbers: [3, 4, 2, 5, 7, 10, 6, 8, 9, 1]
Have you ever wondered how some apps helps you generate the random passwords? Lets see it :
Let’s say you want to generate a random password that consists of a combination of letters, numbers, and special characters. You can use the random module to do this as follows:
import random
import string
# create a sequence of letters, numbers, and special characters
characters = string.ascii_letters + string.digits + string.punctuation
# generate a random password of length 10
password = ''.join(random.choice(characters) for _ in range(10))
print("Random password:", password)
password = ''.join(random.choice(characters) for _ in range(10))
print("Random password 1:", password)
password = ''.join(random.choice(characters) for _ in range(10))
print("Random password 2:", password)
password = ''.join(random.choice(characters) for _ in range(10))
print("Random password 3:", password)
password = ''.join(random.choice(characters) for _ in range(10))
print("Random password 4:", password)
Random password: `G,vqw7ssW
Random password 1: C6&JZBf^;:
Random password 2: BOY$&`M*y_
Random password 3: Nwj+b>vp_@
Random password 4: pcXO?*yq#!
Well, you can modify it according to you also It’s important to note that this is just a basic example and the generated passwords might not be secure enough for real-world use, depending on the security requirements. You should always consult with security experts and follow best practices when generating and storing passwords in your applications.
Difference between “Python random” function and “numpy random” function:
The numpy library also provides a module for random number generation, called numpy.random. This module provides functions similar to those in the random module, but with additional functionality and optimizations for working with arrays and matrices.
Few differences between the random and numpy.random modules:
- Array-based random number generation: numpy.random provides functions for generating random numbers as arrays, whereas the random module generates random numbers as individual values.
For example, numpy.random.rand(5) generates a 1-dimensional array of 5 random values between 0 and 1, while random.random() generates a single random value between 0 and 1.
import random
import numpy as np
# Generating an array of random numbers using numpy.random
random_array = np.random.rand(3, 2)
print("Random array:")
print(random_array)
# Generating an array of random numbers using random
random_list = [random.random() for i in range(6)]
print("Random list:")
print(random_list)
Random array:
[[0.16714623 0.36295139]
[0.58786968 0.66371108]
[0.91193775 0.43411947]]
Random list:
[0.039549229610678194, 0.2895419300587342, 0.39339369809884517, 0.803296015142655, 0.4003648425639498, 0.15103929014608386]
2. Simultaneous random number generation: numpy.random provides functions for generating random arrays in one function call, whereas the random module requires multiple function calls to generate the same number of random numbers.
import numpy as np
# Random float between 0 and 1 using the random module
random_float = random.random()
print("Random float using the random module:", random_float)
# Random float between 0 and 1 using the numpy.random module
random_float = np.random.rand()
print("Random float using the numpy.random module:", random_float)
# Random integer between 0 and 5 using the random module
random_integer = random.randint(0, 6)
print("Random integer using the random module:", random_integer)
# Random integer between 0 and 5 using the numpy.random module
random_integer = np.random.randint(0, 6)
print("Random integer using the numpy.random module:", random_integer)
# Generating a random permutation of the numbers 0 to 5 using the random module
array = [0, 1, 2, 3, 4, 5]
random.shuffle(array)
print("Random permutation using the random module:", array)
# Generating a random permutation of the numbers 0 to 5 using the numpy.random module
array = np.array([0, 1, 2, 3, 4, 5])
random_permutation = np.random.permutation(array)
print("Random permutation using the numpy.random module:", random_permutation)
Random float using the random module: 0.6844371435317264
Random float using the numpy.random module: 0.21534324552047224
Random integer using the random module: 3
Random integer using the numpy.random module: 0
Random permutation using the random module: [3, 1, 0, 5, 2, 4]
Random permutation using the numpy.random module: [0 3 4 2 1 5]
3. Distribution functions: numpy.random provides a number of functions for generating random numbers from specific distributions, such as normal, uniform, and exponential, whereas the random module only provides basic functions for generating random numbers.
import numpy as np
import matplotlib.pyplot as plt
import random
# Generating a random integer between 0 and 100 using the random module
rand_int_random = random.randint(0, 100)
print("Random Integer using random module:", rand_int_random)
# Generating a random integer between 0 and 100 using the numpy.random module
rand_int_numpy = np.random.randint(0, 100)
print("Random Integer using numpy.random module:", rand_int_numpy)
# Generating a random number between 0 and 1 using the random module
rand_float_random = random.random()
print("Random Float using random module:", rand_float_random)
# Generating a random number between 0 and 1 using the numpy.random module
rand_float_numpy = np.random.rand()
print("Random Float using numpy.random module:", rand_float_numpy)
# Generating random numbers from the standard normal distribution
rand_normal = np.random.randn(1000)
# Plotting the histogram of the random numbers
plt.hist(rand_normal, bins=30)
plt.title("Histogram of Random Numbers from the Standard Normal Distribution")
plt.show()
# Generating random numbers from an exponential distribution with lambda=2
rand_exp = np.random.exponential(scale=2, size=1000)
# Plotting the histogram of the random numbers
plt.hist(rand_exp, bins=30)
plt.title("Histogram of Random Numbers from an Exponential Distribution (lambda=2)")
plt.show()
# Generating random numbers from a uniform distribution between 0 and 10
rand_uniform = np.random.uniform(0, 10, 1000)
# Plotting the histogram of the random numbers
plt.hist(rand_uniform, bins=30)
plt.title("Histogram of Random Numbers from a Uniform Distribution between 0 and 10")
plt.show()
Random Integer using random module: 44
Random Integer using numpy.random module: 80
Random Float using random module: 0.5609805611134152
Random Float using numpy.random module: 0.39542963087795047
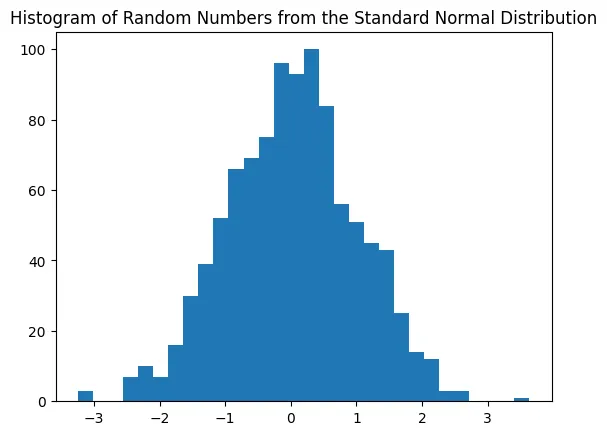
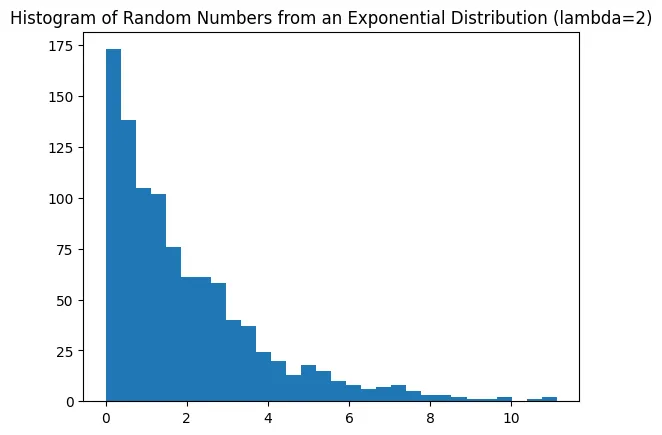


4. Seed management: numpy.random provides functions for setting and getting the random seed, which determines the sequence of random numbers generated, whereas the random module does not have such functions.
import random
import numpy as np
# Setting the seed in the random module
random.seed(10)
rand_int_random = random.randint(0, 100)
print("Random Integer using random module:", rand_int_random)
# Setting the seed in the numpy.random module
np.random.seed(10)
rand_int_numpy = np.random.randint(0, 100)
print("Random Integer using numpy.random module:", rand_int_numpy)
# Setting the seed in the random module again
random.seed(10)
rand_int_random = random.randint(0, 100)
print("Random Integer using random module:", rand_int_random)
# Setting the seed in the numpy.random module again
np.random.seed(10)
rand_int_numpy = np.random.randint(0, 100)
print("Random Integer using numpy.random module:", rand_int_numpy)
Random Integer using random module: 73
Random Integer using numpy.random module: 9
Random Integer using random module: 73
Random Integer using numpy.random module: 9
In general, the numpy.random module is better suited for scientific computing and data analysis tasks that require large arrays of random numbers, while the random module is better for general purpose random number generation. However, both modules can be used for a variety of tasks, depending on your specific needs.
In general, the numpy.random module is better suited for scientific computing and data analysis tasks that require large arrays of random numbers, while the random module is better for general purpose random number generation. However, both modules can be used for a variety of tasks, depending on your specific needs.
— — — — — — — — — — — — — — — — — — — — — — — — — — — — — —
If you like reading stories like this and want to support my writing, Please consider to follow me and igning up to become a Medium member. As a medium member, you have unlimited access to thousands of Python guides and Data science articles.
Do Clap in article if you like it, that will really encourage me.
Thanks
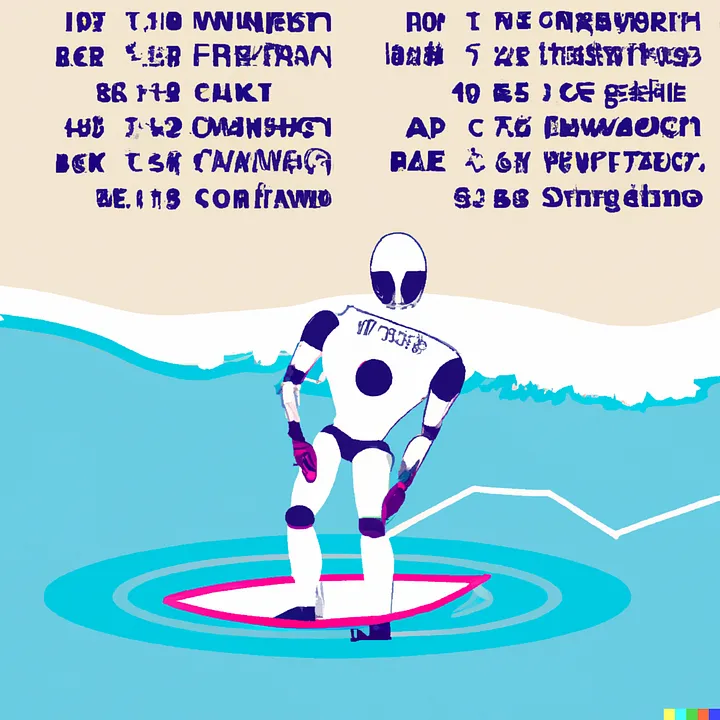
#Python Programming
#Data Science
#Artificial Intelligence
#Machine Learning
#Programming
Leave a Reply
You must be logged in to post a comment.