Why to write long Emails yourself in 2024 ? Lets Create an API in PyQT5

I have created the small interface to show you how you can automate your email writing task, In this example I will use PYQt5 and a very simple interface. To get you API Key, You can follow steps as shown in my another Blog.
You guys can use any interface like flask, django etc to set it also in more beautiful way
Also, I am making it for English and Japanese in case, you guys can change the language parameter in API accordingly to you. I just put japanese as a Example, which i will explain below also
First things first:
install openai first — pip install openai
Below is the code in PyQT5
This script is using the PyQt5 library to create a graphical user interface (GUI) for an email generator that uses the OpenAI API to generate text. The script creates a class called “EmailGenerator” that inherits from the QWidget class, which is a base class for all user interface objects in PyQt5.
- The
__init__
method is called when an instance of the class is created. It sets up the GUI by creating various widgets such as labels, line edits, and buttons. - It also sets up the layout of the widgets using QVBoxLayout and QHBoxLayout.
- The script also sets the api key for OpenAI and sets the default values for max_tokens, n, stop, temperature, and api_key.
- The script also connects the clicked signal of the buttons to the corresponding functions.
- The script also connects the clicked signal of the generate button to the generate_email function.
- The script also calls the show method to display the window on the screen.
- The script also defines several helper functions such as set_max_tokens, set_n, set_stop, set_temperature and set_api_key which are used to set the corresponding variables when the buttons are clicked.
- The script also defines the generate_email function which retrieves the values of the recipient, sender, keywords, topic, and language from the corresponding widgets and uses the OpenAI API to generate an email based on these values.
- Finally, the script sets the text of the result_label to the generated email.
- When the script is run, it creates an instance of the EmailGenerator class, which sets up the GUI and displays it on the screen. The user can then interact with the GUI by entering information into the line edits and selecting options from the combo box.
- By clicking the different buttons, the user can set the max_tokens, n, stop, temperature, and api_key values.
- The user can also generate an email by clicking the “Generate Email” button.
- When the button is clicked, the generate_email function is called and retrieves the values from the line edits and combo box, and sends a request to the OpenAI API using these values.
- The API then generates the email and sends it back to the script.
- The script then sets the text of the result_label to the generated email, which is displayed to the user in the GUI.
It’s important to notice that the script is using the OpenAI API key hardcoded, that’s not a good practice actually, it’s recommended to use a environment variable to store the key. I am just showing it as an example.
import openai
class EmailGenerator(QWidget):
def __init__(self):
super().__init__()
openai.api_key = "your-key"
# Create widgets
self.setWindowTitle("Email Generator by Open AI")
self.resize(800, 400)
self.recipient_label = QLabel("Recipient's name:")
self.recipient_edit = QLineEdit()
self.sender_label = QLabel("Sender's name:")
self.sender_edit = QLineEdit()
self.keywords_label = QLabel("Keywords:")
self.keywords_edit = QLineEdit()
self.topic_label = QLabel("Topic:")
self.topic_edit = QLineEdit()
self.language_label = QLabel("Language:")
self.language_combo = QComboBox()
self.language_combo.addItem("Japanese")
self.language_combo.addItem("English")
self.max_tokens_button = QPushButton("Set max_tokens")
self.n_button = QPushButton("Set n")
self.stop_button = QPushButton("Set stop")
self.temperature_button = QPushButton("Set temperature")
self.api_key_button = QPushButton("Set API Key")
self.generate_button = QPushButton("Generate Email")
self.result_label = QLabel()
self.max_tokens_button.clicked.connect(self.set_max_tokens)
self.n_button.clicked.connect(self.set_n)
self.stop_button.clicked.connect(self.set_stop)
self.temperature_button.clicked.connect(self.set_temperature)
self.api_key_button.clicked.connect(self.set_api_key)
# Create layout and add widgets
self.layout = QVBoxLayout()
self.layout.addWidget(self.recipient_label)
self.layout.addWidget(self.recipient_edit)
self.layout.addWidget(self.sender_label)
self.layout.addWidget(self.sender_edit)
self.layout.addWidget(self.keywords_label)
self.layout.addWidget(self.keywords_edit)
self.layout.addWidget(self.topic_label)
self.layout.addWidget(self.topic_edit)
self.layout.addWidget(self.language_label)
self.layout.addWidget(self.language_combo)
self.layout.addWidget(self.max_tokens_button)
self.layout.addWidget(self.n_button)
self.layout.addWidget(self.stop_button)
self.layout.addWidget(self.temperature_button)
self.layout.addWidget(self.api_key_button)
self.layout.addWidget(self.generate_button)
self.layout.addWidget(self.result_label)
self.setLayout(self.layout)
self.generate_button.clicked.connect(self.generate_email)
self.show()
# Set default values
self.max_tokens = 2048
self.n = 1
self.stop = None
self.temperature = 0.3
self.api_key = "your-key"
def set_max_tokens(self):
# code to set max_tokens value
new_value, ok = QInputDialog.getInt(self, "Set max_tokens", "Enter new value for max_tokens:")
if ok:
self.max_tokens = new_value
pass
def set_n(self):
# code to set n value
new_value, ok = QInputDialog.getInt(self, "Set n", "Enter new value for n:")
if ok:
self.n = new_value
pass
def set_stop(self):
# code to set stop value
new_value, ok = QInputDialog.getText(self, "Set stop", "Enter new value for stop:")
if ok:
self.stop = new_value
pass
def set_temperature(self):
# code to set temperature value
new_value, ok = QInputDialog.getDouble(self, "Set temperature", "Enter new value for temperature:")
if ok:
self.temperature = new_value
pass
def set_api_key(self):
# code to set api key value
new_value, ok = QInputDialog.getText(self, "Set API Key", "Enter new value for API Key:")
if ok:
self.api_key = new_value
pass
def generate_email(self):
recipient_name = self.recipient_edit.text()
sender_name = self.sender_edit.text()
keywords = self.keywords_edit.text()
topic = self.topic_edit.text()
language = self.language_combo.currentText()
if language == "Japanese":
prompt = (f"{sender_name}から{recipient_name}に宛てた『{topic}』についてのメールを、以下のキーワードを使って書いてください: {keywords}")
else:
prompt = (f"Write an email about {topic} addressed to {recipient_name} from {sender_name} using the following keywords: {keywords}")
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=self.max_tokens,
n =self.n,
stop=self.stop,
temperature=self.temperature
)
email_text = response["choices"][0]["text"]
self.result_label.setText(email_text)
In this GUI I have set an default API key in case you do not want to put it everytime, it will still run.
Also the model parameters can be changed as described below and from linked mentioned in below picture

Output GUI :



You can set parameters by clicking on the buttons.
Lets Try another Language:

well its not perfect in japanese, but with input of more exact keyword and adjusting temperature parameter it give better output so that, you can get it according to you.
I have not Provided the complete code thinking its better you guys try it on your preferred UI, but if you guys want a complete code for GUI, drop me a comment asking for it, mentioning your Email, I will send it Personally.
Also I made a API form combine application of Open AI which can do classification, sentiment analysis, code completion etc. I am not willing to share it here so do contact me if you are interested.
Thanks for reading till here.
— — — — — — — — — — — — — — — — — — — — — — — — — — — — — —
If you like reading stories like this and want to support my writing, Please consider to follow me and igning up to become a Medium member. As a medium member, you have unlimited access to thousands of Python guides and Data science articles.
Do Clap in article if you like it, that will really encourage me.
Thanks
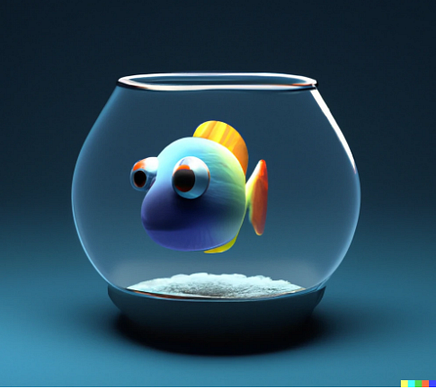
#Artificial Intelligence
#Machine Learning
#OpenAI
#API
#ChatGPT
Leave a Reply
You must be logged in to post a comment.