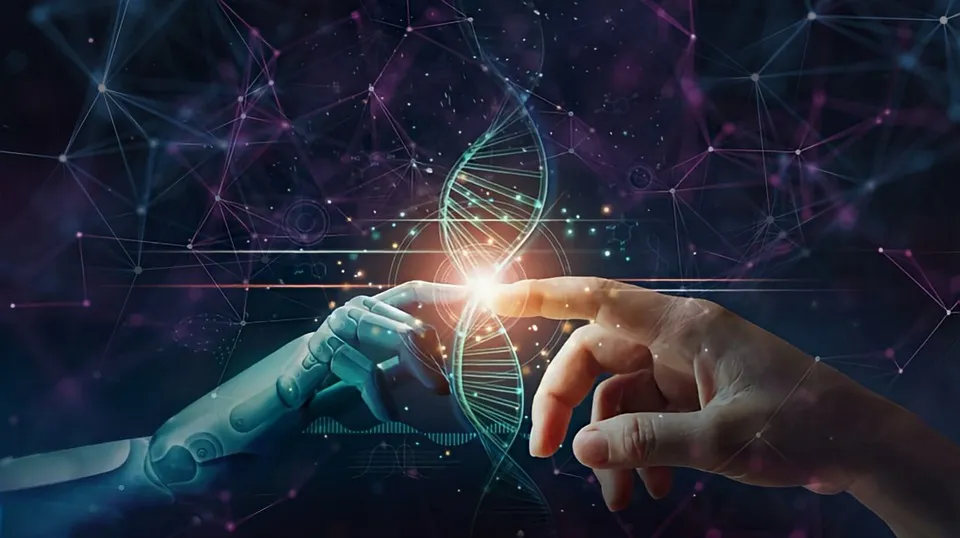
Reinforcement learning (RL) is a type of machine learning where an agent learns to make decisions by interacting with its environment and receiving feedback in the form of rewards or penalties. The agent’s goal is to learn a policy, which is a mapping from states of the environment to actions that the agent can take, that maximizes the cumulative reward over time.
Reinforcement learning is different from supervised learning in that it does not rely on labeled data to learn. Instead, the agent must explore its environment and learn from its own experiences. This exploration can be done in a number of ways, such as through trial-and-error or by using techniques like Monte Carlo Tree Search.
The learning process in RL is called trial and error learning, where an agent takes actions in an environment and receives rewards or penalties. The agent learns from this feedback by updating its policy so as to take actions that will lead to higher rewards in the future. The agent uses a value function to estimate the expected cumulative reward from each state or state-action pair, which is used to guide its decision making.
Reinforcement learning has been successfully applied in a variety of domains, such as robotics, game playing, and autonomous vehicles. It has also been used to solve complex problems in fields like finance and energy management. RL is a powerful technique for training agents to make decisions in complex, dynamic environments and can lead to the development of intelligent and autonomous systems.
The mechanism of reinforcement learning can be broken down into several key components:
- Environment: The environment is the system that the agent interacts with. It can be a physical system, like a robot, or a simulated system, like a game. The environment defines the states that the agent can be in, the actions that the agent can take, and the rewards or penalties that the agent receives as a result of its actions.
class TicTacToeEnv:
def __init__(self):
self.board = np.zeros((3, 3))
self.player = 1
def check_game_over(self):
for i in range(3):
if np.all(self.board[i, :] == self.player) or np.all(self.board[:, i] == self.player):
return True
if np.all(np.diag(self.board) == self.player) or np.all(np.diag(np.fliplr(self.board)) == self.player):
return True
if np.count_nonzero(self.board) == 9:
return True
return False
def make_move(self, row, col):
if self.board[row, col] == 0:
self.board[row, col] = self.player
if self.check_game_over():
return self.player
if self.player == 1:
self.player = 2
else:
self.player = 1
return 0
else:
return -1
2. Agent: The agent is the system that learns to make decisions. It receives observations of the current state of the environment and decides which action to take. The agent uses its policy, which is a mapping from states to actions, to make these decisions.
class Agent:
def __init__(self, num_states, num_actions):
self.num_states = num_states
self.num_actions = num_actions
self.policy = np.ones((num_states, num_actions)) / num_actions
self.value_function = np.zeros(num_states)
def choose_action(self, state):
return np.random.choice(self.num_actions, p=self.policy[state, :])
def update_policy(self, state, action, new_state, reward, alpha=0.1):
self.value_function[state] += alpha * (reward + self.value_function[new_state] - self.value_function[state])
self.policy[state, action] +=
3. Policy: The policy is a function that the agent uses to decide which action to take in a given state. It maps from states to actions, and it can be deterministic or stochastic. The agent updates its policy as it learns from its experiences.
class Agent:
def __init__(self, num_states, num_actions):
self.num_states = num_states
self.num_actions = num_actions
self.policy = np.ones((num_states, num_actions)) / num_actions
self.value_function = np.zeros(num_states)
def choose_action(self, state):
return np.random.choice(self.num_actions, p=self.policy[state, :])
def update_policy(self, state, action, new_state, reward, alpha=0.1):
self.value_function[state] += alpha * (reward + self.value_function[new_state] - self.value_function[state])
self.policy[state, action] += alpha * (reward - self.value_function[state]) * (1 - self.policy[state, action])
4. Value function: The value function is a prediction of the expected cumulative reward that the agent will receive in the future, starting from a given state or state-action pair. The agent uses the value function to guide its decision making by selecting actions that will lead to higher expected rewards.
class Agent:
def __init__(self, num_states, num_actions):
self.num_states = num_states
self.num_actions = num_actions
self.policy = np.ones((num_states, num_actions)) / num_actions
self.value_function = np.zeros(num_states)
def update_value_function(self, state, new_state, reward, gamma=0.9):
self.value_function[state] += alpha * (reward + gamma * self.value_function[new_state] - self.value_function[state])
5. Reinforcement signal: The reinforcement signal, also called reward signal, is the feedback that the agent receives from the environment after taking an action. It is a scalar value that the agent uses to evaluate the quality of its actions. The agent’s goal is to learn a policy that maximizes the cumulative reward over time.
def train(self, env, num_episodes=1000):
for episode in range(num_episodes):
state = env.reset()
done = False
while not done:
action = self.choose_action(state)
new_state, reward, done = env.step(action)
self.update_policy(state, action, new_state, reward)
self.update_value_function(state, new_state, reward)
state = new_state
In the code examples that I provided, the class Agent
is used to represent the learning agent that interacts with the environment. The class contains the agent’s policy, value function, and other related parameters such as the learning rate and discount factor. The Agent class has various methods such as choose_action
and update_policy
and update_value_function
which are used to implement the Q-learning algorithm.
The reason that I used class Agent
is to show how the different mechanisms of RL can be modularized and organized into reusable objects in order to make the code more readable and maintainable. The class contains all the functionality that the agent needs to interact with the environment and learn from its experiences, such as choosing actions, updating its policy and value function, etc.
The class-based approach allows for easy modification of the agent’s behavior by modifying the methods of the class, as well as easy instantiation of multiple agents with different configurations. It also enables separating the agent’s logic from the environment logic, this way the environment can be use with different agents without modifying the environment code.
One example of a real-world application of reinforcement learning is the use of RL to train agents that can play video games. RL has been used to train agents that can play games like Pong, Breakout, and Space Invaders with superhuman performance. Here is an example of how an RL agent can be trained to play the game Pong using the OpenAI Gym library:
import gym
import numpy as np
# create the environment
env = gym.make("Pong-v0")
# define the Q-table and other parameters
q_table = np.zeros((6400, 6))
alpha = 0.1
gamma = 0.99
epsilon = 0.1
# helper function to discretize the state space
def discretize_state(state):
state = state[35:195]
state = state[::2, ::2, 0]
state[state == 144] = 0
state[state == 109] = 0
state[state != 0] = 1
return state.ravel().astype(int)
# helper function to select an action using epsilon-greedy policy
def select_action(state, q_table, epsilon):
if np.random.uniform(0, 1) < epsilon:
return env.action_space.sample()
else:
return np.argmax(q_table[state, :])
for episode in range(10000):
# reset the environment and get the initial state
state = discretize_state(env.reset())
done = False
while not done:
# select an action using epsilon-greedy policy
action = select_action(state, q_table, epsilon)
# take the action and observe the next state and reward
next_state, reward, done, _ = env.step(action)
next_state = discretize_state(next_state)
# update the Q-table
q_table[state, action] += alpha * (reward + gamma * np.max(q_table[next_state, :]) - q_table[state, action])
# set the new state as the current state
state = next_state
# decrease the epsilon value over time
epsilon -= 2e-7
the agent starts by creating an environment using the OpenAI Gym library and initializing the Q-table and other parameters. It then repeatedly interacts with the environment by selecting actions, observing rewards, and updating the Q-table using the Q-learning algorithm. The agent discretizes the state space using a helper function and chooses an action using an epsilon-greedy policy. The agent continues playing the game until it reaches a maximum number of episodes or a certain stopping criteria.
As you can see, the agent is trained to play Pong by the combination of Q-Learning and OpenAI-gym. However, training an agent with RL is computationally expensive and time-consuming, it also can be very hard to converge for many problems.
This is just one example of how RL can be used to train agents for real-world tasks. RL has also been used to train agents for robotics, finance, and many other applications.
Real Life Applications :
Reinforcement learning (RL) has been used in a variety of real-world applications, some of which include:
- Robotics: RL has been used to train robots to perform a variety of tasks such as object manipulation, grasping, and navigation. RL algorithms have been used to train robots to learn from their mistakes and adapt to changes in their environment, which makes them more robust and flexible than traditional rule-based approaches.
- Autonomous vehicles: RL has been used to train self-driving cars to make decisions such as when to accelerate, brake, and turn. RL algorithms have been used to train cars to handle different driving scenarios such as merging on to a highway, overtaking other vehicles, and dealing with unexpected obstacles.
- Finance: RL has been used to develop trading algorithms for the stock market. RL algorithms have been used to train agents to make decisions about buying and selling stocks based on historical data and current market conditions.
- Energy Management: RL has been used to optimize energy consumption in buildings and data centers. RL algorithms have been used to learn the energy consumption patterns of different devices and adjust their usage accordingly to minimize energy costs while maintaining comfort and performance.
- Healthcare: RL has been used to develop decision support systems in the healthcare field. RL algorithms have been used to train models to predict the progression of a disease, or identify the best treatment plan for a patient based on their medical history and current health status.
- Game Playing: One of the early successes of RL has been in the field of game playing, RL has been used to train agents to play various games such as chess, go, dota 2 and others.
- Advertising: RL has been used to optimize the performance of online advertising systems. RL algorithms have been used to train agents to decide which ads to display to different users based on their browsing history, demographics, and other factors.
- Chat GPT-3: chatbots powered by GPT (Generative Pre-trained Transformer) is a good example of an application of reinforcement learning (RL) in the real world.GPT-based chatbot models are pre-trained on a large corpus of text data using unsupervised learning techniques such as language modeling. After pre-training, the model can be fine-tuned on a smaller dataset of conversational data to make it perform a specific task such as answering questions or engaging in a conversation.During the conversation, the GPT-based chatbot uses the learned conversational patterns from the fine-tuned dataset to generate appropriate responses. Additionally, the chatbot can use reinforcement learning techniques like Q-learning or SARSA to improve its performance over time by learning from the rewards (or penalties) it receives based on the quality of its responses.One of the advantages of using GPT-based chatbots is that they can generate human-like responses, they can also understand the context of the conversation and adjust their responses accordingly. They are used in a variety of fields such as customer service, virtual assistants and also in entertainment like creating AI writers to create stories, music and even jokes.Overall, GPT-based chatbot is an example of how advanced machine learning techniques such as RL can be used to create powerful AI-powered conversational systems that can improve human-computer interaction

— — — — — — — — — — — — — — — — — — — — — — — — — — — — — — — —
Leave a Reply
You must be logged in to post a comment.