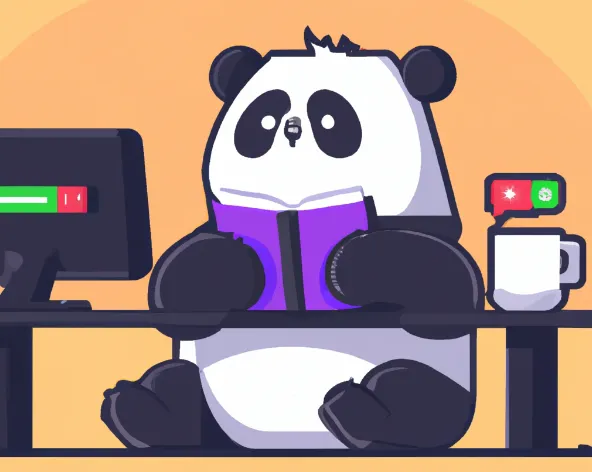
NumPy is a powerful and flexible Python library that is used for scientific computing and data analysis. It provides a wide range of functions and tools that allow you to work with large, multi-dimensional arrays and matrices of numerical data, as well as to perform mathematical operations on these data.
One of the main advantages of NumPy is its ability to efficiently perform element-wise operations on large arrays and matrices. This makes it an ideal choice for tasks such as linear algebra, statistical analysis, and signal processing, where you need to perform operations on large amounts of data quickly and efficiently.
NumPy also includes a range of functions for generating random numbers, as well as for performing statistical and mathematical operations on arrays. This makes it a useful tool for data simulation and machine learning tasks.
In addition to its numerical capabilities, NumPy also provides support for working with missing data and handling data types. It includes a range of functions for reading and writing data to and from files, as well as for working with data stored in different formats.
NumPy is a powerful and versatile library that is widely used in the scientific and data analysis communities. It provides a range of functions and tools that allow you to work efficiently with large, multi-dimensional arrays and matrices of numerical data, and to perform a wide range of mathematical and statistical operations on these data.
Learning NumPy can be a useful and rewarding experience, as it is a powerful tool for scientific computing and data analysis. Here are some suggestions for how to get started with learning NumPy:
- Install NumPy: To start using NumPy, you will first need to install it. You can install NumPy using the
pip
package manager by running the following command:pip install numpy
- Familiarize yourself with basic NumPy concepts: NumPy is based on the concept of arrays, which are similar to lists but are more efficient for certain types of operations. It is also important to understand the difference between NumPy arrays and Python lists. You can learn more about these concepts by reading the NumPy documentation or by working through a tutorial.
- Practice using NumPy: Once you have a basic understanding of NumPy, it is helpful to practice using it by working through some examples and exercises. There are many resources online that provide examples and exercises for learning NumPy, including tutorials, online courses, and notebooks.
- Explore advanced NumPy features: NumPy includes many advanced features that are useful for more advanced tasks, such as linear algebra, statistical analysis, and machine learning. As you become more comfortable with NumPy, you can explore these features in more depth to expand your knowledge and skills.
Here are a few basic functions of NumPy, along with examples of how to use them:
array
: This function allows you to create a NumPy array from a list or tuple.
For example:
import numpy as np
# Create a NumPy array from a list
arr = np.array([1, 2, 3, 4, 5])
print(arr)
# Output: [1 2 3 4 5]
# Create a NumPy array from a tuple
arr = np.array((6, 7, 8, 9, 10))
print(arr)
# Output: [6 7 8 9 10]
zeros
: This function creates an array of a given shape filled with zeros.
For example:
import numpy as np
# Create a 2x3 array of zeros
arr = np.zeros((2, 3))
print(arr)
# Output: [[0. 0. 0.]
# [0. 0. 0.]]
ones
: This function creates an array of a given shape filled with ones. For example:
import numpy as np
# Create a 2x3 array of ones
arr = np.ones((2, 3))
print(arr)
# Output: [[1. 1. 1.]
# [1. 1. 1.]]
arange
: This function creates an array of evenly spaced values within a given range. For example:
import numpy as np
# Create an array with values from 0 to 9
arr = np.arange(10)
print(arr)
# Output: [0 1 2 3 4 5 6 7 8 9]
# Create an array with values from 10 to 20, with a step size of 2
arr = np.arange(10, 21, 2)
print(arr)
# Output: [10 12 14 16 18 20]
linspace
: This function creates an array of evenly spaced values within a given range. The function takes three arguments: the start value, the end value, and the number of values you want to generate.
For example:
import numpy as np
# Create an array with 5 values evenly spaced between 0 and 1
arr = np.linspace(0, 1, 5)
print(arr)
# Output: [0. 0.25 0.5 0.75 1. ]
shape
: This function returns the shape of an array as a tuple of integers.
For example:
import numpy as np
# Create a 2x3 array
arr = np.array([[1, 2, 3], [4, 5, 6]])
# Get the shape of the array
shape = arr.shape
print(shape)
# Output: (2, 3)
reshape
: This function returns an array with the same data but a different shape. It takes a tuple as an argument, representing the new shape of the array.
For example:
import numpy as np
# Create a 1D array with 6 elements
arr = np.arange(6)
print(arr)
# Output: [0 1 2 3 4 5]
# Reshape the array into a 2x3 array
arr = arr.reshape((2, 3))
print(arr)
# Output: [[0 1 2]
# [3 4 5]]
transpose
: This function returns an array with the axes transposed.
For example:
import numpy as np
# Create a 2x3 array
arr = np.array([[1, 2, 3], [4, 5, 6]])
print(arr)
# Output: [[1 2 3]
# [4 5 6]]
# Transpose the array
arr = arr.transpose()
print(arr)
# Output: [[1 4]
# [2 5]
# [3 6]]
NumPy can be used in combination with the Pandas library to perform various operations on dataframes.
Here are a few examples of commonly used NumPy functions when working with dataframes:
mean
: This function calculates the mean value of an array or a column in a dataframe. For example:
import pandas as pd
import numpy as np
# Read a CSV file into a dataframe
df = pd.read_csv("data.csv")
# Calculate the mean value of the "Value" column
mean_value = np.mean(df["Value"])
print(mean_value)
std
: This function calculates the standard deviation of an array or a column in a dataframe.
For example:
import pandas as pd
import numpy as np
# Read a CSV file into a dataframe
df = pd.read_csv("data.csv")
# Calculate the standard deviation of the "Value" column
std_value = np.std(df["Value"])
print(std_value)
corrcoef
: This function calculates the correlation coefficient between two arrays or two columns in a dataframe.
For example:
import pandas as pd
import numpy as np
# Read a CSV file into a dataframe
df = pd.read_csv("data.csv")
# Calculate the correlation coefficient between the "Value" and "Weight" columns
corr_coef = np.corrcoef(df["Value"], df["Weight"])[0, 1]
print(corr_coef)
unique
: This function returns the unique values of an array or a column in a dataframe.
For example:
import pandas as pd
import numpy as np
# Read a CSV file into a dataframe
df = pd.read_csv("data.csv")
# Get the unique values of the "Category" column
unique_categories = np.unique(df["Category"])
print(unique_categories)
These are just a few examples of the many NumPy functions that can be used in combination with the Pandas library to perform various operations on dataframes.
There is much more to learn anout Numpy and I will come upwith more information about Numpy in Details in my next blogs.
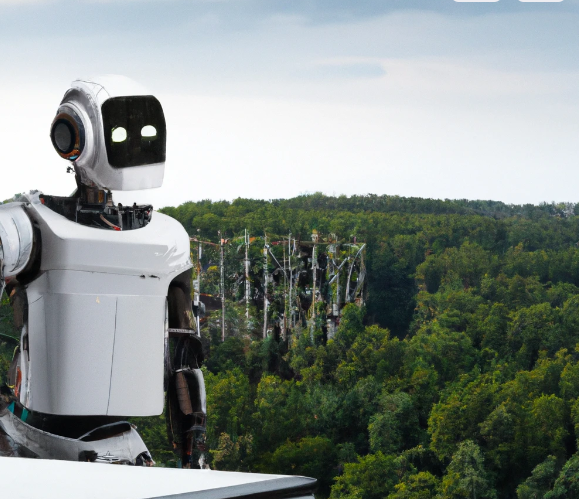
#Numpy
#Beginners Guide
#Towards Data Science
#Artificial Intelligence
#Machine Learning
1 Pingback