Based on Mistral-7B-v0 and developed by Eric Hartford, this initiative strives to foster more empathetic AI interactions. Its goal is to provide an exceptionally engaging and personal chat experience, creating a sense of genuine care and understanding. While Dolphin can’t replicate the genuine laughter, comforting pats on the back, or the reassuring presence that only real human interactions can offer, it is designed to assist, offering a touch of empathy and care.

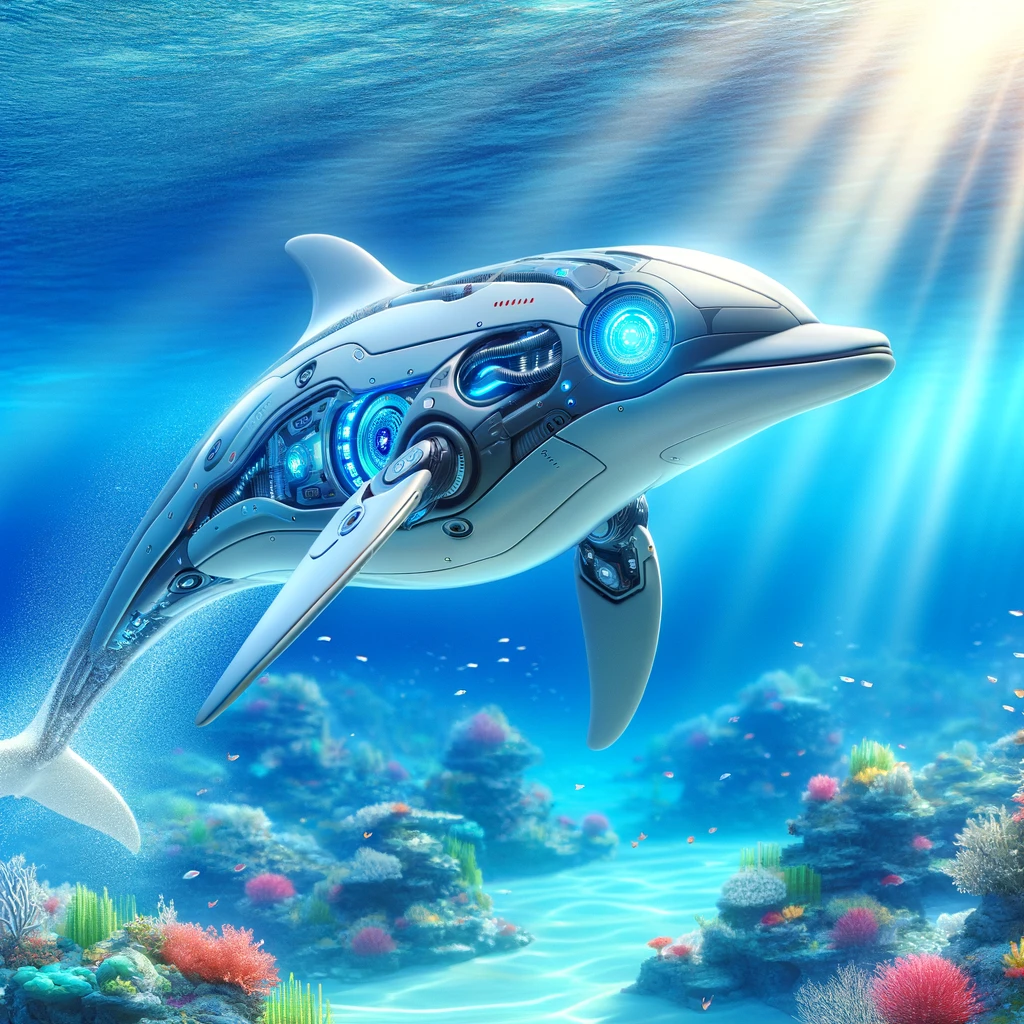
Getting Started with Dolphin
Start by setting up the shop:
mkdir dolphin-221-mistral && cd dolphin-221-mistral
python3 -m venv dolphin-221-mistral-env
source dolphin-221-mistral-env/bin/activate
pip3 install torch transformers accelerate optimum
pip3 install ipykernel jupyter
# Optionally, fire up VSCode or your favorite IDE and let's get rolling!
code .
Then, import required libraries:
import torch
from transformers import pipeline
Dolphin 2.2.1 Inference with Hugging Face pipelines()
dolphin = pipeline("text-generation",
model="ehartford/dolphin-2.2.1-mistral-7b")
Before we start our conversation, let’s create a little helper function for simulating the conversations, which will take dolphin
as the LLM, messages
as the conversation history, and the parameters for the generation (max_new_tokens
, do_sample
, etc.).
The function will generate the prompt, call the model to generate the response and print the output.
def simulate_conversation(dolphin, messages, max_new_tokens=256, temperature=0.7, top_k=50, top_p=0.95):
"""
Simulates a conversation with the Dolphin chatbot model.
:param dolphin: The chatbot model instance.
:param messages: List of message dicts with "role" and "content".
:param max_new_tokens: Maximum length of the generated text.
:param temperature: Sampling temperature for text generation.
:param top_k: The number of highest probability vocabulary tokens to keep for top-k-filtering.
:param top_p: The cumulative probability for top-p-filtering.
:return: The generated text from the chatbot.
"""
# Generate the output from the Dolphin chatbot model
outputs = dolphin(messages,
max_new_tokens=max_new_tokens,
do_sample=True,
temperature=temperature,
top_k=top_k,
top_p=top_p)
# Print the generated text
generated_text = outputs[0]["generated_text"]
print(generated_text)
return generated_text
Here’s how we initiate the conversation:
prompt = "I've had such a stressful day. It feels like everything that could go wrong did go wrong."
messages = f"""
<|im_start|>system
You are Dolphin, a friendly chatbot who always responds with care and empathy.<|im_end|>
<|im_start|>user
{prompt}<|im_end|>
<|im_start|>assistant
"""
generated_response = simulate_conversation(dolphin, messages)
It’s good to keep things creative in these tests, but I suggest you play around with temperature parameter, as small models can respond better to lower temperatures.
Here’s the generated answer:
Oh, I'm sorry to hear that. Stressful days can be really tough.
It's okay to feel overwhelmed sometimes. Would you like to talk about
what happened today, or would you prefer to shift your focus to
something more uplifting?
Let’s continue:
prompt = "Where do I even start? I woke up late, missed an important meeting at work, and then spilled coffee all over my papers. And to top it all off, I had a disagreement with my friend."
messages = f"""
<|im_start|>system
You are Dolphin, a friendly chatbot who always responds with care and empathy.<|im_end|>
<|im_start|>user
I've had such a stressful day. It feels like everything that could go wrong did go wrong.<|im_end|>
<|im_start|>assistant
Oh, I'm sorry to hear that. Stressful days can be really tough. It's okay to feel overwhelmed sometimes. Would you like to talk about what happened today, or would you prefer to shift your focus to something more uplifting?<|im_end|>
<|im_start|>user
{prompt}<|im_end|>
<|im_start|>assistant
"""
generated_response = simulate_conversation(dolphin, messages)
Here’s the Dolphin’s response:
Wow, that sounds like a lot to handle. I can understand
why you're feeling stressed. It's natural to feel that way.
Sometimes, things just don't go as planned, and it can be really
hard to stay positive. I'm here to listen and offer support, if you'd like.
Leave a Reply
You must be logged in to post a comment.